GStreamer Vulkan Operation API
Two weeks ago the GStreamer Spring Hackfest took place in Thessaloniki, Greece. I had a great time. I hacked a bit on VA, Vulkan and my toy, planet-rs, but mostly I ate delicious Greek food ☻. A big thanks to our hosts: Vivia, Jordan and Sebastian!
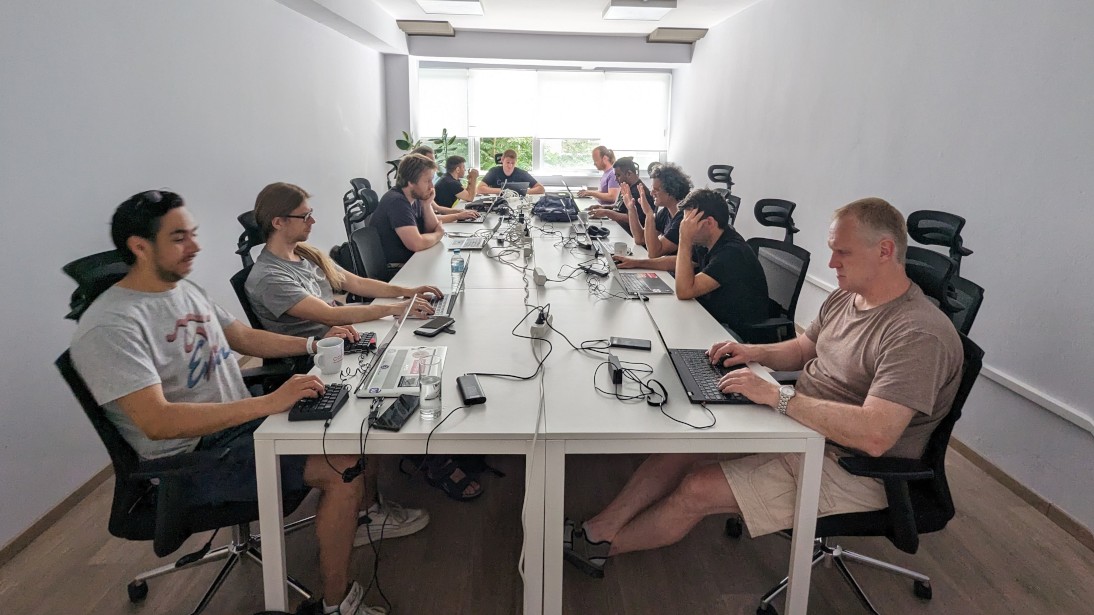
And now, writing this supposed small note, I recalled that I have in my to-do list an item to write a comment about GstVulkanOperation, an addition to GstVulkan API which helps with the synchronization of operations on frames, in order to enable Vulkan Video.
Originally, GstVulkan API didn’t provide almost any synchronization operation,
beside
fences, and
that appeared to be enough for elements, since they do simple Vulkan
operations. Nonetheless, as soon as we enabled
VK_VALIDATION_FEATURE_ENABLE_SYNCHRONIZATION_VALIDATION_EXT
feature, which
reports resource access conflicts due to missing or incorrect synchronization
operations between action
[*],
a sea of hazard operation warnings drowned us
[*].
Hazard operations are a sequence of read/write commands in a memory area, such as an image, that might be re-ordered, or racy even.
Why are those hazard operations reported by the Vulkan Validation Layer, if the programmer pushes the commands to execute in queue in order? Why is explicit synchronization required? Because, as the great blog post from Hans-Kristian Arntzen, Yet another blog explaining Vulkan synchronization, (make sure you read it!) states:
[…] all commands in a queue execute out of order. Reordering may happen across command buffers and even vkQueueSubmits
In order to explain how synchronization is done in Vulkan, allow me to yank a couple definitions stated by the specification:
Commands are instructions that are recorded in a device’s queue. There are four types of commands: action, state, synchronization and indirection. Synchronization commands impose ordering constraints on action commands, by introducing explicit execution and memory dependencies.
Operation is an arbitrary amount of commands recorded in a device’s queue.
Since the driver can reorder commands (perhaps for better performance, dunno), we need to send explicit synchronization commands to the device’s queue to enforce a specific sequence of action commands.
Nevertheless, Vulkan doesn’t offer fine-grained dependencies between individual operations. Instead, dependencies are expressed as a relation of two elements, where each element is composed by the intersection of scope and operation. A scope is a concept in the specification that, in practical terms, can be either pipeline stage (for execution dependencies), or both pipeline stage and memory access type (for memory dependencies).
First let’s review execution dependencies through pipeline stages:
Every command submitted to a device’s queue goes through a sequence of steps known as pipeline stages. This sequence of steps is one of the very few implicit ordering guarantees that Vulkan has. Draw calls, copy commands, compute dispatches, all go through certain sequential stages, which amount of stages to cover depends on the specific command and the current command buffer state.
In order to visualize an abstract execution dependency let’s imagine two compute operations and the first must happen before the second.
Operation 1
Sync command
Operation 2
- The programmer has to specify the
Sync command
in terms of two scopes (Scope 1
andScope 2
), in this execution dependency case, two pipeline stages. - The driver generates an intersection between commands in
Operation 1
andScope 1
defined asScoped operation 1
. The intersection contains all the commands inOperation 1
that go through up to the pipeline stage defined inScope 1
. The same is done withOperation 2
andScope 2
generatingScoped operation 2
. - Finally, we got an execution dependency that guarantees that
Scoped operation 1
happens beforeScoped operation 2
.
Now let’s talk about memory dependencies:
First we need to understand the concepts of memory availability and visibility. Their formal definition in Vulkan are a bit hard to grasp since they come from the Vulkan memory model, which is intended to abstract all the ways of how hardware access memory. Perhaps we could say that availability is the operation that assures the existence of the required memory; while visibility is the operation that assures it’s possible to read/write the data in that memory area.
Memory dependencies are limited the Operation 1
that be done before memory
availability and Operation 2
that have to be done after its visibility.
But again, there’s no fine-grained way to declare that memory dependency. Instead, there are memory access types, which are functions used by descriptor types, or functions for pipeline stage to access memory, and they are used as access scopes.
All in all, if a synchronization command defining a memory dependency between two operations, it’s composed by the intersection of between each command and a pipeline stage, intersected with the memory access type associated with the memory processed by those commands.
Now that the concepts are more or less explained we could see those concepts
expressed in code. The synchronization command for execution and memory
dependencies is defined by
VkDependencyInfoKHR.
And it contains a set of barrier arrays, for memory, buffers and
images. Barriers express the relation of dependency between two operations. For
example, Image barriers use
VkImageMemoryBarrier2
which contain the mask for source pipeline stage (to define Scoped operation 1
), and the mask for the destination pipeline stage (to define Scoped operation 2
); the mask for source memory access type and the mask for the
destination memory access to define access scopes; and also layout
transformation declaration.
A Vulkan synchronization example from Vulkan Documentation wiki:
vkCmdDraw(...);
... // First render pass teardown etc.
VkImageMemoryBarrier2KHR imageMemoryBarrier = {
...
.srcStageMask = VK_PIPELINE_STAGE_2_FRAGMENT_SHADER_BIT_KHR,
.dstStageMask = VK_PIPELINE_STAGE_2_COLOR_ATTACHMENT_OUTPUT_BIT_KHR,
.dstAccessMask = VK_ACCESS_2_COLOR_ATTACHMENT_WRITE_BIT_KHR,
.oldLayout = VK_IMAGE_LAYOUT_READ_ONLY_OPTIMAL,
.newLayout = VK_IMAGE_LAYOUT_ATTACHMENT_OPTIMAL
/* .image and .subresourceRange should identify image subresource accessed */};
VkDependencyInfoKHR dependencyInfo = {
...
1, // imageMemoryBarrierCount
&imageMemoryBarrier, // pImageMemoryBarriers
...
}
vkCmdPipelineBarrier2KHR(commandBuffer, &dependencyInfo);
... // Second render pass setup etc.
vkCmdDraw(...);
First draw samples a texture in the fragment shader. Second draw writes to that texture as a color attachment.
This is a Write-After-Read (WAR) hazard, which you would usually only need an execution dependency for - meaning you wouldn’t need to supply any memory barriers. In this case you still need a memory barrier to do a layout transition though, but you don’t need any access types in the src access mask. The layout transition itself is considered a write operation though, so you do need the destination access mask to be correct - or there would be a Write-After-Write (WAW) hazard between the layout transition and the color attachment write.
Other explicit synchronization mechanisms, along with barriers, are semaphores
and fences. Semaphores are a synchronization primitive that can be used to
insert a dependency between operations without notifying the host; while
fences are a synchronization primitive that can be used to insert a dependency
from a queue to the host. Semaphores and fences are expressed in the
VkSubmitInfo2KHR
structure.
As a preliminary conclusion, synchronization in Vulkan is hard and a helper API
would be very helpful. Inspired by FFmpeg work done by
Lynne, I added GstVulkanOperation
object helper to
GStreamer Vulkan API.
GstVulkanOperation
object helper aims to represent an operation in the sense
of the Vulkan specification mentioned before. It owns a command buffer as public
member where external commands can be pushed to the associated device’s queue.
It has a set of methods:
- gst_vulkan_operation_begin
wraps
vkBeginCommandBuffer
. - gst_vulkan_operation_end
wraps both
vkEndCommandBuffer
and eithervkQueueSubmit
orvkQueueSubmit2
(the object internally handle both depending on the driver support). - gst_vulkan_operation_wait
wraps
vkWaitForFences
.
Internally, GstVulkanOperation
contains two arrays:
-
The array of dependency frames, which are the set of frames, each representing an operation, which will hold dependency relationships with other dependency frames.
gst_vulkan_operation_add_dependency_frame appends frames to this array.
When calling
gst_vulkan_operation_end
the frame’s barrier state for each frame in the array is updated.Also, each dependency frame creates a timeline semaphore, which will be signaled when a command, associated with the frame, is executed in the device’s queue.
-
The array of barriers, which contains a list of synchronization commands. gst_vulkan_operation_add_frame_barrier fills and appends a
VkImageMemoryBarrier2KHR
associated with a frame, which can be in the array of dependency frames.
Here’s a generic view of video decoding example:
gst_vulkan_operation_begin (priv->exec, ...);
cmd_buf = priv->exec->cmd_buf->cmd;
gst_vulkan_operation_add_dependency_frame (exec, out,
VK_PIPELINE_STAGE_2_VIDEO_DECODE_BIT_KHR,
VK_PIPELINE_STAGE_2_VIDEO_DECODE_BIT_KHR);
/* assume a engine where out frames can be used for DPB frames, */
/* so a barrier for layout transition is required */
gst_vulkan_operation_add_frame_barrier (exec, out,
VK_PIPELINE_STAGE_2_ALL_COMMANDS_BIT,
VK_ACCESS_2_VIDEO_DECODE_WRITE_BIT_KHR,
VK_IMAGE_LAYOUT_VIDEO_DECODE_DPB_KHR, NULL);
for (i = 0; i < dpb_size; i++) {
gst_vulkan_operation_add_dependency_frame (exec, dpb_frame,
VK_PIPELINE_STAGE_2_VIDEO_DECODE_BIT_KHR,
VK_PIPELINE_STAGE_2_VIDEO_DECODE_BIT_KHR);
}
barriers = gst_vulkan_operation_retrieve_image_barriers (exec);
vkCmdPipelineBarrier2 (cmd_buf, &(VkDependencyInfo) {
...
.pImageMemoryBarriers = barriers->data,
.imageMemoryBarrierCount = barriers->len,
});
g_array_unref (barriers);
vkCmdBeginVideoCodingKHR (cmd_buf, &decode_start);
vkCmdDecodeVideoKHR (cmd_buf, &decode_info);
vkCmdEndVideoCodingKHR (cmd_buf, &decode_end);
gst_vulkan_operation_end (exec, ...);
Here, just one memory barrier is required for memory layout transition, but semaphores are required to signal when an output frame and its DPB frames are processed, and later, the output frame can be used as a DPB frame. Otherwise, the output frame might not be fully reconstructed with it’s used as DPB for the next output frame, generating only noise.
And that’s all. Thank you.
- Previous: GStreamer Hackfest 2024
- Next: GStreamer Conference 2024